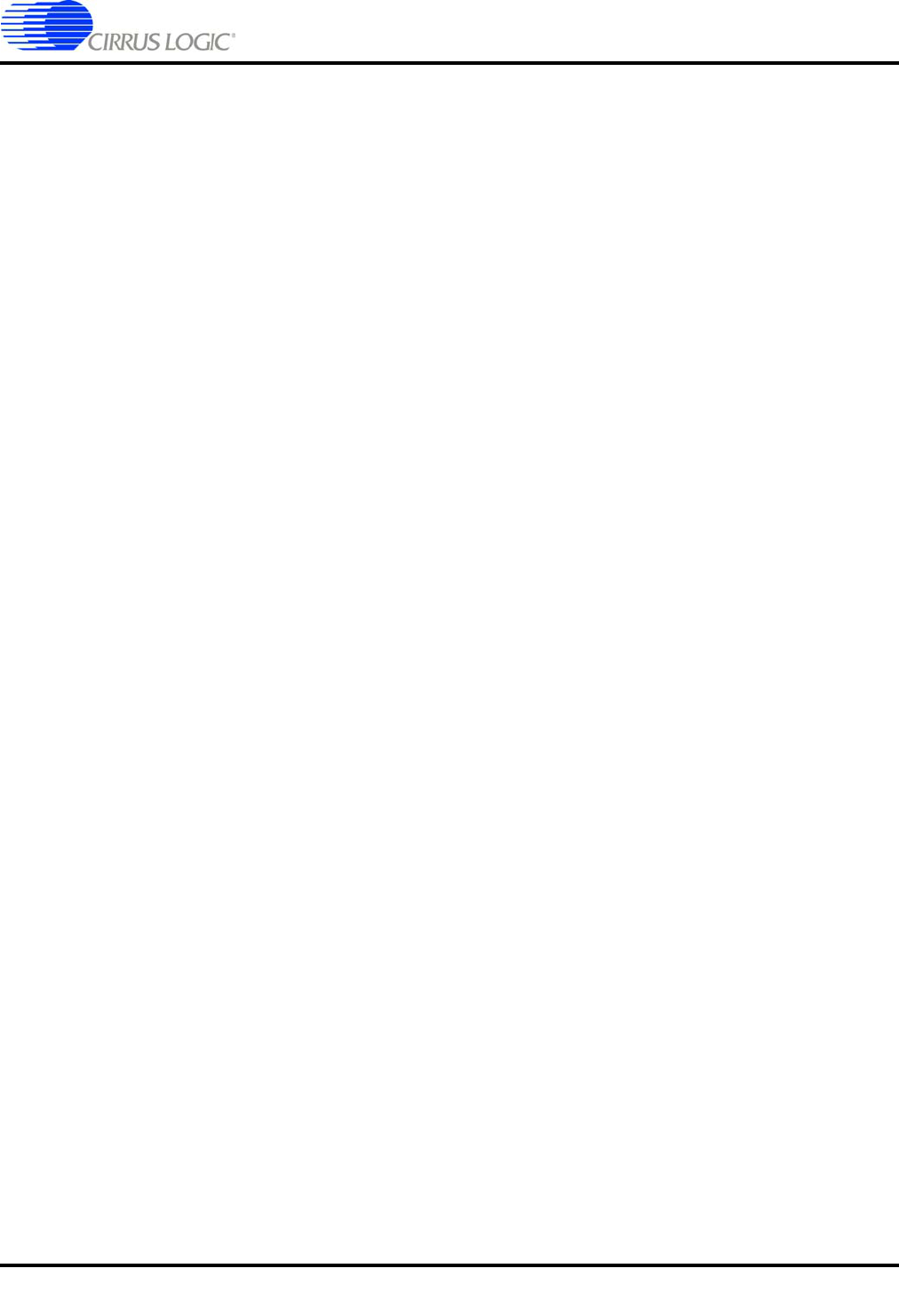
CobraNet Hardware User’s Manual
HMI Reference Code
DS651UM23 ©Copyright 2005 Cirrus Logic, Inc. 35
Version 2.3
while( !( ReadRegister( MSG_D ) & ( 1 << MSG_TRANSLATION_BO ) ) );
/* goto translation */
WriteRegister( MSG_C, MOP_GOTO_TRANSLATION_READ );
SendMessage( CVR_MULTIPLEX_OP );
/* "garbage" read clears data pipeline */
ReadRegister( DATA_D );
/* maintain local pointers */
PeekPointer = PokePointer = address;
PeekLimit = PokeLimit = PeekPointer +
ReadRegister( MSG_C ) + ( ReadRegister( MSG_B ) << 8 );
/* read-only region addressed */
if( !( ReadRegister( MSG_A ) & ( 1 << MSG_WRITABLE_BO ) ) ) {
PokeLimit = PokePointer;
}
}
unsigned long Peek(
long address )
{
if( address != PeekPointer ) {
SetAddress( address );
}
if( PeekPointer >= PeekLimit ) {
throw "Peek addressing error!";
}
unsigned long value = ReadRegister( DATA_A ) << 24;
value += ReadRegister( DATA_B ) << 16;
value += ReadRegister( DATA_C ) << 8;
value += ReadRegister( DATA_D );
PeekPointer++; /* maintain local pointer */
return value;
}
void Poke(
long address,
unsigned long value )
{
if( address != PokePointer ) {
SetAddress( address );
}
if( PokePointer >= PokeLimit ) {
throw "Poke addressing error or read-only!";
}
WriteRegister( DATA_A, (unsigned char) ( ( value >> 24 ) & 0xff ) );
WriteRegister( DATA_B, (unsigned char) ( ( value >> 16 ) & 0xff ) );
WriteRegister( DATA_C, (unsigned char) ( ( value >> 8 ) & 0xff ) );
WriteRegister( DATA_D, (unsigned char) ( value & 0xff ) );
/* maintain local pointers */
PokePointer++;
PeekPointer = -1; /* force SetAddress()next Peek() to freshen data */
}